Webhook authentication Copy section link Copied!
Since your notification server will be publicly accessible, AgoraPay includes an authentication mechanism so you can verify that incoming events have been genuinely created by AgoraPay.
For additional verification:
The public exit IP range is 158.190.51.32/27, which is the same in both the approval and production environments.
HMAC control Copy section link Copied!
Each webhook notification event contains Http headers in addition to the JSON body (Body-Request).
1 2 3 4 5 6 7 |
|
We are going to focus on the "Authorization" HTTP header that includes the following fields, separated by "/".
Fields
Description
Version
The current version of the authorisation header is "hmac 1.0"
Nonce
This is a 36-character random data type uuid v4.
Timestamp
A timestamp in seconds since 1/1/1970 UTC (linux time).
Key Id
This is the identifier for the key generated when the notification account was created.
HMAC
Computed HMAC.
HMAC control example Copy section link Copied!
Let's take the following event received from a POST request on the webhook URL "https://YourMarketPlace.fr/webhook", containing the following HTTP header and JSON payload body (Body-Request) :
1 2 3 4 5 6 7 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
The following fields need to be extracted from the authorisation header:
Field name
Value
version
hmac 1.0
nonce
08b72fcf-97e8-4a54-866b-dad9ea7f57b7
timestamp
1722427893459
keyid
00934d0f-8993-4be6-96c2-b9c2d76acec5
hmac
CBFABE34D6A05EC6072E7E93C5DF206DBFDCCE6E60BACDB83BFACB60FC41B43E
The marketplace must perform the following verifications on the extracted information:
- Check the HMAC control version is the same as the one you implemented
- Check that the keyid has the same value as your marketplace key ID (given by your implementation manager)
- The HMAC you compute corresponds to the computed HMAC passed in the authorisation header,
Compute Hmac Copy section link Copied!
To compute the HMAC, you need to:
- Compute the SHA-256 hash of the "Request-Body" and Uppercase it:
1 |
|
- Create the plain to text string:
$authorizationElements[1] = nonce
$authorizationElements[2] = timeStamp
1 |
|
POST;https://YourMarketPlace.fr/webhook;F2C72B386EDA769A19224E6EF049CB3DDA756E09E31EE5C16E3F90005BB5C7FA;08b72fcf-97e8-4a54-866b-dad9ea7f57b7;1722427893459
- Compute the HMAC-SHA-256 hash, using the $plainTextToComputeHmac and your marketplace Hook HMAC Key (given by your implementation manager) and capitalise it.
1 |
|
Notice how the "pack" function is being called on the HMAC key given by your implementaion manager
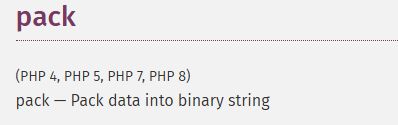
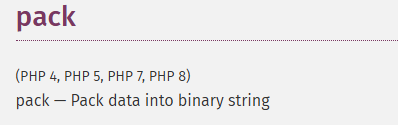
Full PHP function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
Other HMAC calculation implementations Copy section link Copied!
Here are some other code examples of the HMAC control algorithm:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
|